Whenever you upload an image to your WordPress site through the media library, it automatically creates and stores multiple additional versions of that image. If your site doesn’t utilize these extra image sizes, they will consume valuable storage space and increase the size of your server backups.
In this guide, I’ll walk you through the default image sizes, explain why they are generated, show you how to identify additional image sizes and provide you with the code snippet to prevent WordPress from creating unnecessary image sizes.
Don’t feel like reading? Skip to the snippet or the plugin.
Default Image Sizes in WordPress
Here is a table of all the default image sizes in WordPress:
As you can see there are quite a lot!
This means that each time you upload an image to your WordPress site, it could generate and store up to 7 additional image versions on your server! And these are only the default sizes defined in core, it doesn’t include any extra sizes defined by your themes & plugins.
Image Sizes Created by Your WordPress Theme & Plugins
Most classic WordPress themes (non-block themes) don’t make use of the thumbnail, medium and large core sizes. They usually register their own image sizes that work best with the design and layout of the theme.
Plugins may also register new image sizes. This is common in plugins used to display posts or include custom post types. Prime examples would be directory, events, real estate, ecommerce, LMS, page builders, etc.
This means your site could be creating even more image sizes and really bloating up your server and backup files.
It’s a good idea to read the documentation for the theme and all plugins used on your site to ensure they are not creating unnecessary resized versions of your images. Most well coded themes and plugins will offer a setting to modify or disable any additional image sizes.
If you are using our Total theme be sure to check out the documentation regarding the Image Sizes Panel. If you are not, but you are a developer you may want to have a look at how I did things for inspiration.
Later in this guide I will provide you with an easy code-based solution for viewing all registered image sizes on your site.
Before explaining how to stop WordPress from generating alternative image sizes for your uploads, it’s important to understand why WordPress creates these extra sizes in the first place. Below is a list of the key reasons:
Faster Loading Times: Smaller images load faster.
Responsive Images: Provide alternative image sizes for different screen sizes.
Consistency: For keeping all your featured images the same size.
High-Resolution Displays: Provide alternative images for high-resolution and retina displays.
Featured Thumbnails: Provide alternative image sizes for use in different parts of the site or with theme/plugins specific elements.
Server Performance: As in the case with the -scaled image as noted in the table above.
When logged into your WordPress site if you go to Settings > Media you will find options to set three of the core WordPress image sizes (Thumbnail, Medium, Large).
Setting the width and height of all fields to 0 will prevent WordPress from creating those extra images. This is the first thing I do whenever I install WordPress and recommend it for most sites.
However, this isn’t a full solution for preventing unwanted resized images from your site. Keep reading to find out more and see my code snippet for disabling all image sizes.
The Big Image Size Threshold in WordPress
In WordPress 5.3.0 they introduced what is known as the Big Image Size Threshold. This is the maximum width or height an image can be for which WordPress will generate additional image sizes when the image is uploaded. The default value is 2560.
If an image is uploaded to the media library that exceeds the threshold, WordPress will scale down the image and it will be used as the largest available size. WordPress will then generate all the additional image sizes defined on your site based on the scaled down version.
So, if you are uploading images that are larger than 2560 (width or height) WordPress will create extra images on your server. You may want to disable or adjust this accordingly.
Disable the Big Image Size Threshold
If you want to be able to upload large images to your site without WordPress scaling them down and creating an alternative version you can do so with the following code snippet:
// Disable the big image size threshold
add_filter( ‘big_image_size_threshold’, ‘__return_false’ );
Caution: The big image threshold exists for a reason and I highly recommend NOT disabling it. Disabling this feature could cause performance issues on the server. If you know only smaller images are uploaded to the site than you can disable it, but it won’t matter anyway to leave it on.
Modify the Big Image Size Threshold
If your site needs to allow for larger images (for example a photography website) then you may want to modify the big image size threshold value. This way WordPress won’t scale down your images.
Here is an example of how you can change the value from 2560 to 5000:
// Modify the big image size threshold
add_filter( ‘big_image_size_threshold’, function( $threshold ) {
return 5000;
} );
How to Check what Image Sizes are Defined on Your Site?
Unfortunately WordPress doesn’t have a native way of seeing a list of all the image sizes registered on your website. There are plugins you can use for this, but since I focus primarily on code based tutorials I will show you how you can use code to display a list of registered images on your site.
Quick & “Dirty” Method
If you copy and paste this code into your functions.php file then refresh your site you will see a list of the registered image sizes at the top of the live site. You can then copy and paste them into a text file for reference then remove the code.
// Display all defined image sizes at the top of the site inside a <pre> tag
add_action( ‘wp_head’, function() {
echo ‘<pre>’;
foreach ( (array) wp_get_registered_image_subsizes() as $size => $dims ) {
$width = $dims[‘width’] ?? 0;
$height = $dims[‘height’] ?? 0;
echo “{$size}: {$width}x{$height}n”;
}
echo ‘</pre>’;
} );
Display Registered Image Sizes in the WP Admin
Having access to a list of registered image sizes in your WordPress admin panel is ideal. This way if you ever enable a new plugin or switch themes you can quickly check to see if new image sizes are being defined.
The following code snippet will display a table of all defined image sizes at the bottom of the Settings > Media panel:
// Add a table of image sizes to the Settings > Media admin page
add_action( ‘admin_init’, function() {
add_settings_section(
‘dummy_registered_image_sizes_info’,
esc_html__( ‘Registered Image Sizes’, ‘text_domain’ ),
function() {
echo ‘<table class=”wp-list-table widefat fixed striped”>’;
echo ‘<thead><tr><th>’ . esc_html__( ‘Name’, ‘text_domain’ ) . ‘</th><th>’ . esc_html__( ‘Dimensions’, ‘text_domain’ ) . ‘</th></tr></thead>’;
foreach ( (array) wp_get_registered_image_subsizes() as $size => $dims ) {
if ( ! in_array( $size, [ ‘thumbnail’, ‘medium’, ‘large’ ], true ) ) {
$width = $dims[‘width’] ?? 0;
$height = $dims[‘height’] ?? 0;
echo “<tr><td><strong>{$size}</strong></td><td>{$width}x{$height}</td>”;
}
}
echo ‘</table>’;
},
‘media’
);
}, PHP_INT_MAX );
Technically we are defining a new settings section with this code but we aren’t actually registering any settings. Instead we set the callback for our section to return a table that loops through and displays all registered image sizes.
Here is a screenshot of the end result:
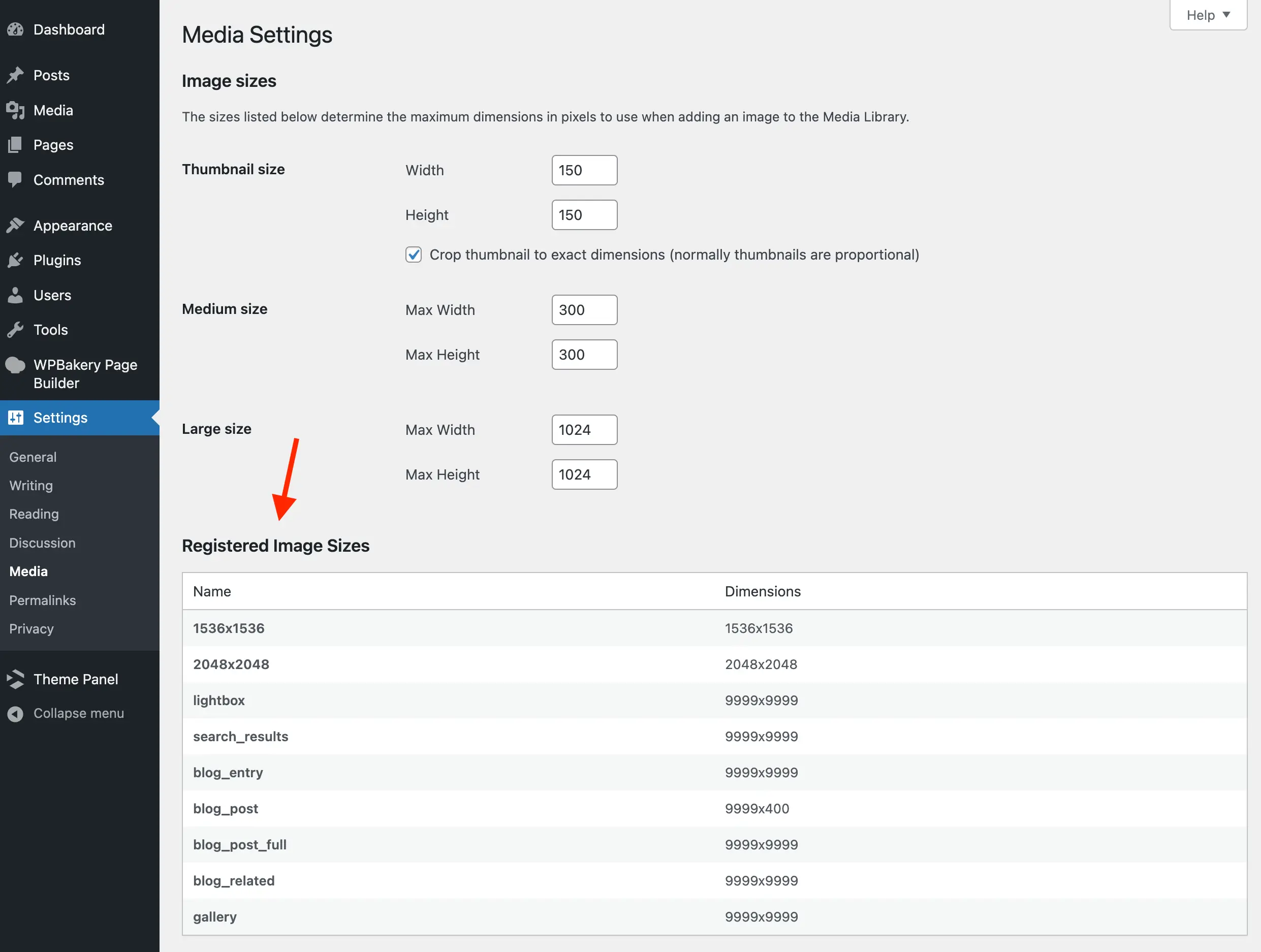
This example is from the Total theme where the default theme registered image sizes are set to 9999×9999 which is very large so they wont ever crop by default.
There isn’t any option you can just check in the WordPress admin so in order to stop WordPress from creating cropped versions of your images you will need to use a little code. Luckily it can be done with a single line of code!
// Return false for calculated resized image dimensions
add_filter( ‘image_resize_dimensions’, ‘__return_false’ );
To understand how this code works we’ll take a look at the core image_resize_dimensions() function. It’s quite long, so I won’t post it here, but you can click on the previous link if you want to see all the code associated with the function.
Basically, whenever WordPress is going to create a new image size it uses this function to return the calculated resize dimensions for the image, which it then passes to the WP_Image_Editor class. Hooking into the image_resize_dimensions filter and returning false will make the function exit early so no calculations are made and ultimately no extra image will be generated.
Optimized Code Snippet
The previous snippet will prevent WordPress from cropping any image when a new image size is requested. This will work regardless of when an image size is requested.
However, we can optimize our code by hooking into the intermediate_image_sizes_advanced filter which returns the array of image sizes automatically generated when uploading an image.
// Return an empty list of image sizes to generate on upload
add_filter( ‘intermediate_image_sizes_advanced’, ‘__return_empty_array’ );
By returning an empty array for the filter we let WordPress know there shouldn’t be any extra images generated when uploading a new image to our site. Now, whenever you upload an image to your website only the original image will be added to the server.
Full Snippet:
Here are both snippets combined:
// Return false for calculated resized image dimensions
add_filter( ‘image_resize_dimensions’, ‘__return_false’ );
// Return an empty list of image sizes to generate on upload
add_filter( ‘intermediate_image_sizes_advanced’, ‘__return_empty_array’ );
Now, if you are hooking into intermediate_image_sizes_advanced you don’t necessarily have to also hook into image_resize_dimensions.
The reason for hooking into both filters is in case a theme or plugin is using it’s own “on-the-fly” cropping solution – which hopefully makes use of the image_resize_dimensions() function.
WP Disable All Image Sizes Plugin
I’ve also put the code snippet into a little plugin if you want to just download and install it instead. This plugin should never require any updates and the WordPress plugin review process is a nightmare so for now I’m just leaving it on Github.
WP Disable all Image Sizes Plugin Github Repository
The plugin will do 3 things:
Disable the big image size threshold.
Return false for the image_resize_dimensions filter.
Return an empty array for the intermediate_image_sizes_advanced filter.
If you’ve added the code snippet to your site and find that image sizes are still being generated when you upload images, you will need to disable plugins and/or your theme to locate the culprit.
As mentioned previously, it’s possible there is a custom”on-the-fly” image cropping solution on your site that is not using core WP functionality and thus the core filters won’t affect it.
Exclude Specific Image Sizes from Being Created on Upload
Perhaps you don’t want to prevent all image sizes from being generated. It’s possible to modify the code to only exclude certain image sizes, like such:
// Exclude certain image sizes from being generated on upload
add_filter( ‘intermediate_image_sizes_advanced’, function( $sizes ) {
$sizes_to_exclude = [
‘thumbnail’,
‘medium’,
‘large’,
‘medium_large’,
‘1536×1536’,
‘2048×2048’,
];
foreach ( $sizes_to_exclude as $size_to_exclude ) {
unset( $sizes[ $size_to_exclude ] );
}
return $sizes;
} );
If you want to exclude only certain image sizes make sure you are NOT hooking into image_resize_dimensions and returning false.
And you probably noticed I included the thumbnail, medium and large image sizes in the snippet. This way, even if someone messes with the settings in the admin those image sizes will be excluded.
Tips for Reducing the Need for Resized Images
At the beginning of the article I gave some of the reasons as to why WordPress creates additional image sizes. With those in mind, if you are planning on disabling the extra image sizes here are some tips to ensure you don’t create extra “issues”.
Don’t Upload Massive Images: Make sure you are not uploading massive images to your site. If you don’t have control over this, make sure you don’t remove the big image threshold and be ok with the fact that your site will create scaled images when needed.
Upload Big “Enough” Images: It’s hard to say how big is big enough as it depends on the site and context in which the image is added. But, you will want your images to be large enough that they look good on high-resolution screens while being small enough (in kb) that it doesn’t slow down site loading.
Optimize Images Prior to Upload: There are many great image optimization plugins out there, but why bloat up your site and consume server resources if you can optimize your images prior to upload. I personally use tinypng.com and convert my images to webP format prior to upload.
Use Image Aspect Ratios: One of the main reasons image sizes are created is to keep a consistent look across your posts as all your featured images will be cropped to the same dimensions. Rather, you can use the CSS aspect-ratio property to target your images.
These are the main tips that come to my mind, let me know in the comments if you have some other good suggestions or concerns that should be addressed.
How to Remove Old Image Sizes from Your Server
Adding the code to prevent WordPress from creating extra image sizes will only take affect for newly uploaded images. If you are adding the code to an existing site there may be tons of old cropped images on the server you will want to clean up.
There are several methods you can use to delete old resized images and many blogs recommend using CLI (terminal) – however, WordPress stores image sizes in the attachment meta data so I don’t recommend that solution.
The easiest method I’ve found is using the Force Regenerate Thumbnails plugin. You can enable the plugin, run the process and then delete it from your site.
The plugin works by looping through every image attachment on the site, pulling it’s defined sizes from the meta data and deleting them all. After deleting the image sizes it runs the core wp_generate_attachment_metadata() function which will re-create the intermediate image sizes for the attachment. So, if you’ve disabled extra image sizes via the previous code no images will be generated.
I could provide you with a code snippet to delete old image sizes from your site, however, the process can be very resource intensive and is best done using AJAX. The Force Regenerate Thumbnails plugin will go through each image at a time and if there are any issues it will log and display them.
The plugin will also show you what images were deleted and generated which is really nice!
As always, before you install any new plugins or delete anything from your site you should make sure you have a full backup. I’ve used the plugin many times without issues, but it’s better safe than sorry.
Conclusion
Personally I think disabling all extra image sizes is best for most websites. It ensures your server space is not consumed by images (many which will never be used) and in turn keeps your backups significantly smaller.
There could be an argument also for SEO. I don’t know much about Google Image Search Optimization, but perhaps having many of the same image at different sizes could cause issues. If you are an SEO expert, please let me know in the comments if that’s the case!
Further reading:
You may also be interested in the following: